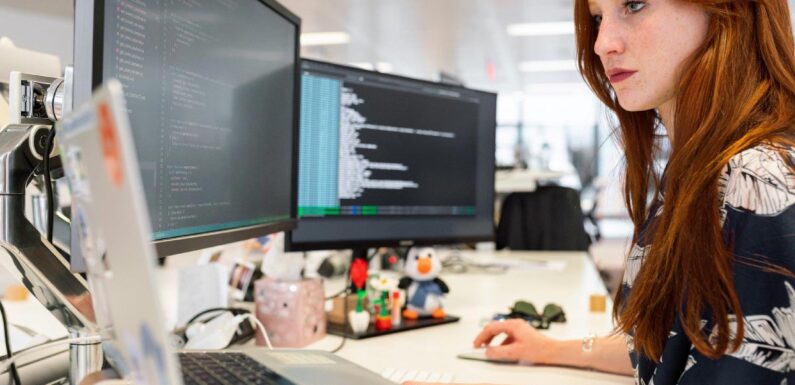
API testing is essential for verifying that the APIs your application relies on are functioning as expected. In this guide, we’ll introduce you to REST Assured, a popular tool for API testing. We’ll show you how to use the REST Assured Framework to verify the correctness and completeness of your API responses.
What is REST Assured?
REST Assured is a Java library that makes it easy to test RESTful web services. It provides a simple DSL (domain-specific language) for describing the desired behavior of RESTful web service and automatically verifies the results.
REST Assured can be used for both automated and manual testing. It has been designed to work with popular open-source automation frameworks such as Selenium, Cucumber, and Spock. REST Assured is particularly well suited for API testing, but it can also be used for testing other types of web services. REST Assured is open source, released under the Apache License 2.0.
How to Use?
REST Assured can be used in a number of ways. The most common way is to use it as a Java library, which can be added to your project using Maven or Gradle. Alternatively, you can use REST Assured as a standalone executable JAR file. Finally, you can use REST Assured from the command line using the curl utility.
Once you have REST Assured set up, you can start writing tests. The first step is to create a RestAssured object, which will act as the entry point for all of your tests. Next, you need to specify the URL of the web service you want to test. Finally, you can add one or more verification steps, such as assert that() or body().
REST Assured also supports a number of other features, such as parameterization, authentication, and proxying. For more information, see the REST Assured framework documentation.
API testing with REST Assured: An Introduction
API testing is the process of verifying that an API (Application Programming Interface) functions as intended. API tests can be used to verify the correctness of data returned by an API, ensure that APIs meet performance requirements, and detect and diagnose problems with APIs.
API testing is different from other types of software testing, such as GUI testing or unit testing. GUI tests verify the functionality of a user interface, while unit tests verify the functionality of individual units of code. API tests focus on the interactions between different units of code, rather than on the individual units themselves.
API testing can be performed manually, or it can be automated. Automated API tests are typically faster and more reliable than manual tests, and they can be run more often. However, automated tests require more setup and maintenance than manual tests.
There’s a good chance you’re at least somewhat familiar with the REST Assured Framework by now. But do you know how to get started using it? Let’s walk through a simple example of how to write tests using the REST Assured Framework. We’ll also discuss some of the benefits of using this framework for your API testing needs.
In this example, we’ll be testing a simple JSON-based API that returns weather data for a given location. The API we’ll be using is the OpenWeatherMap API, which is a free and open-source weather API.
First, let’s take a look at the endpoint we’ll be testing. The OpenWeatherMap API has a number of different endpoints, but for this example, we’ll start by defining a few constants that we’ll need for our test.
“`
const ENDPOINT = “api.openweathermap.org/data/”;
const API_KEY = “your-api-key”;
const CITY = “London”;
Next, we’ll create we’ll be using the “Current Weather Data” endpoint. This endpoint takes a city name as a parameter and returns data for that city.
Now that we know what endpoint we’ll be testing, let’s take a look at how to write a test using REST Assured.
REST Assured tests are written in Java, so you’ll need to have a basic understanding of the language before you can get started. But don’t worry – we’ll walk you through everything you need to know.
First, we’ll create a new class called WeatherTest and annotate it with @Test. This annotation tells REST Assured that this is a test class and that it should run the tests in this class.
@Test
public class WeatherTest {
Next, we’ll create a new method called testWeatherData and annotate it with @Test. This annotation tells REST Assured that this is a test method and that it should run this method as a test.
@Test
public void testWeatherData() {
In this method, we’ll use the given() method to specify the endpoint we want to test. We’ll also use the query param() method to specify the city parameter.
Given().
queryParam(“q”, CITY).
We’ll use the when() method to send a GET request to the endpoint.
When().
get(ENDPOINT).
Finally, we’ll use the then() method to assert that the response code is equal to 200. This assertion verifies that the request was successful and that the data was returned successfully.
Then().
assertThat().
statusCode(200);
}
}
“`
Now that we’ve written our test, let’s run it to see if it passes. To do this, simply right-click on the WeatherTest class and select Run As > JUnit Test.
If everything goes well, you should see a green bar indicating that the test has passed. Congratulations! You’ve just written your first REST Assured test. Let’s hop on to the benefits of using the REST Assured framework.
The Benefits :
1. Automated API testing for faster, more reliable releases
2. Improved collaboration and communication across teams
3. Easier debugging and troubleshooting of issues
4. Greater confidence in the quality of your APIs
5. REST Assured is a Java library that makes API Testing easy and fun. It allows you to easily send requests to APIs and examine the responses.
6. REST Assured also has a wide variety of features that make API Testing easier, such as assertions, filters, and custom headers.
7. REST Assured has a large user community and is well supported.
8. It is open source and released under the Apache License 2.0.
9. REST Assured integrates well with popular automation frameworks, such as Selenium and Cucumber.
10. You can use REST Assured from the command line using the curl utility.
To Conclude :
We’ve just scratched the surface of what’s possible with REST Assured. This was a simple guide beneficial to most of the API Testers. To learn more, stay tuned for more blog posts on API testing!
Happy testing!